Psycopg2.operationalerror: ssl connection has been closed unexpectedly
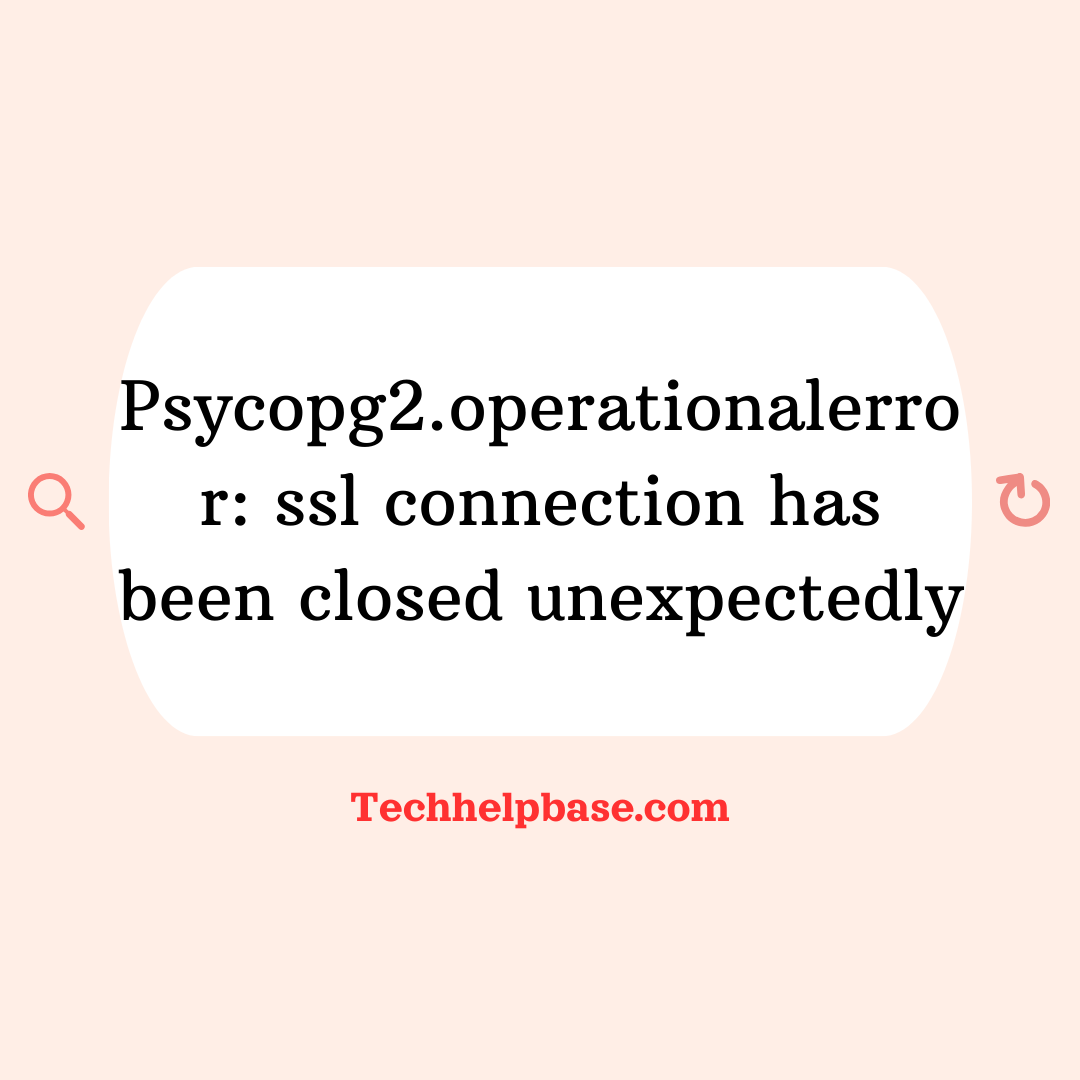
When working with PostgreSQL databases in Python using the popular library psycopg2, you may encounter an error like this: psycopg2.operationalerror: ssl connection has been closed unexpectedly. This issue can be frustrating, especially when it interrupts your database operations and leads to unexpected behavior. Let’s break down what this error means, what causes it, and how you can resolve it.
What is psycopg2 OperationalError?
Psycopg2 is a widely used PostgreSQL adapter for Python, enabling developers to connect and interact with PostgreSQL databases efficiently. When you see the error psycopg2.operationalerror: ssl connection has been closed unexpectedly, it indicates a problem in maintaining a secure connection to the PostgreSQL server over SSL (Secure Socket Layer).
This error often arises when there’s a disruption in the SSL connection, either because the server closed the connection unexpectedly or due to a failure in the client-side configuration. It manifests for users when their database queries suddenly fail, or the database connection closes prematurely, often without clear warning or error messages beforehand.
Possible Causes of psycopg2 OperationalError: SSL Connection Closed
Understanding the root causes of this error is crucial for troubleshooting. Several factors can contribute to the psycopg2.operationalerror: ssl connection has been closed unexpectedly:
- Network Interruptions: A common cause of this issue is an unstable network, especially when there’s a brief outage or latency spike. The SSL connection between the client and the PostgreSQL server may time out or be forcibly closed due to network instability.
- Database Server Configuration: Some PostgreSQL servers may have strict SSL requirements or misconfigurations that lead to connection closure. This could involve certificate issues, outdated SSL protocols, or mismatches between the server and client-side SSL configurations.
- Firewall Settings: If there is a firewall between your client and the PostgreSQL server, it could terminate long-standing connections due to inactivity or misconfigured timeout settings.
- Idle Connection Timeouts: Some hosting providers or PostgreSQL server configurations automatically close idle connections after a set period. If your connection remains idle for too long, it may get terminated by the server.
- Incorrect SSL Parameters: If you’re using invalid or improperly configured SSL certificates, you may run into the psycopg2.operationalerror: ssl connection has been closed unexpectedly error. This is particularly true when dealing with self-signed certificates or when the certificate authority (CA) is not recognized.
Real-World Examples
Developers often run into this error in various scenarios. For example, a user on a forum shared that they frequently encountered this error when running long queries on a cloud-hosted PostgreSQL instance. After several minutes, the query would fail, and the error would be thrown. Another user reported intermittent disconnections when using a VPN, as the network instability caused their SSL connections to drop unexpectedly.
These examples highlight that while the error is technical in nature, the cause is often a combination of factors, including network, configuration, and server settings.
How to Fix psycopg2 OperationalError: SSL Connection Has Been Closed Unexpectedly
Now that we understand the possible causes, let’s walk through a step-by-step guide to resolving this issue. Multiple troubleshooting methods can be applied depending on the specific scenario you’re facing.
Step 1: Check Your Network Connection
Since network instability is one of the primary causes, the first step is to ensure you have a stable and reliable network connection. If you’re using a VPN, try disconnecting it and running your database queries without it. Similarly, if you’re connected over Wi-Fi, try switching to a wired connection to reduce network latency.
Step 2: Configure SSL Properly
Ensure that your SSL configuration on both the client and server sides is correct. For example:
- Make sure that the PostgreSQL server is configured to accept SSL connections (
ssl = on
in thepostgresql.conf
file). - Check that your client application is using the correct SSL mode. You can set this by specifying
sslmode
when establishing a connection. Common modes include:require
: Ensures that SSL is used for the connection.verify-ca
orverify-full
: Verifies the certificate authority (CA) of the server. If you’re using self-signed certificates, ensure that the CA is trusted on your client machine.
A typical connection string might look like this:
conn = psycopg2.connect(
dbname="your_db",
user="your_user",
password="your_password",
host="your_host",
sslmode="require"
)
Adjust these parameters to ensure SSL is correctly configured and your certificates are valid.
Step 3: Adjust PostgreSQL Server Timeout Settings
If you suspect that idle connection timeouts are the issue, you can increase the timeout settings on the PostgreSQL server. Check the values of parameters such as:
tcp_keepalives_idle
tcp_keepalives_interval
tcp_keepalives_count
These settings control how often the server checks whether the connection is still active. Increasing the intervals between these checks can prevent the server from closing the connection prematurely.
You can adjust these settings in your postgresql.conf
file:
tcp_keepalives_idle = 300 # Time in seconds before idle connection is checked
tcp_keepalives_interval = 60 # Time in seconds between keepalive checks
tcp_keepalives_count = 5 # Number of keepalive messages sent before disconnect
Step 4: Investigate Firewall Rules
Firewalls can sometimes close idle connections or connections with long-standing queries. Check if there are any firewall rules in place that might be interrupting the connection between your client and the PostgreSQL server.
If you suspect this might be the case, consult with your network administrator or cloud provider to either adjust the firewall settings or whitelist the IP addresses involved.
Step 5: Update psycopg2 and PostgreSQL
Another potential fix is updating your software stack. The psycopg2 library and PostgreSQL server both receive frequent updates to fix bugs and improve SSL handling. Updating to the latest version of psycopg2 and PostgreSQL can sometimes resolve connection issues, including the psycopg2.operationalerror: ssl connection has been closed unexpectedly.
Run the following command to update psycopg2:
pip install --upgrade psycopg2
Similarly, ensure your PostgreSQL server is up-to-date by following the appropriate upgrade steps for your operating system or cloud provider.
How to Prevent This Issue in the Future
Once you’ve resolved the psycopg2.operationalerror: ssl connection has been closed unexpectedly, there are a few best practices you can follow to minimize the chances of encountering this issue again:
- Monitor Your Network: Use network monitoring tools to track connection stability, especially when working with databases over the internet. Tools like Pingdom or Datadog can alert you to network issues before they affect your database connections.
- Configure Keepalives: Ensure that both client and server-side keepalive settings are properly configured. This can prevent the connection from being closed due to inactivity.
- Use Connection Pooling: Implement connection pooling to manage your database connections efficiently. Tools like
pgbouncer
can help manage and reuse connections, reducing the chances of idle timeouts or disconnections. - Update Regularly: Keeping your PostgreSQL server and psycopg2 library up to date can protect you from bugs and connection issues related to outdated SSL protocols or certificates.